After learning how to get started with LOVE2D and make Hello World with LOVE2D, I wanted to make a minimal but playable game.
I went to the official LOVE2D website and went to see some tutorials. I chose to try any one game from the Simple Game Tutorial website. I chose the Bird game from there. This is a simple version of the Flappy Bird game. Since there is a box instead of a bird, I am calling it Flappy Box. Yeah, I know it isn't flapping either. But cut the box some slack please. :D
I skimmed through the tutorial. It was pretty neat and well explained. Instead of giving the full code at once, it guides you through each step by adding/removing/updating over previous codes.
And yes, I too went through all the steps thoroughly. I promise! ;)
I got confused in the first few steps when it said to add new codes. It was actually replacing some parts in the function with new codes. So, watch out for that.
Here's an example.
In the next step:
So you can see that it's the same function. So, instead of adding the code given above completely into the file, you are expected to update the previous function love.draw().
Here, -- etc. refers to the previous lines of codes in the same function.
So, the final code will actually be the sum of two.
function love.draw() -- code for drawing background love.graphics.setColor(.14, .36, .46) love.graphics.rectangle('fill', 0, 0, 300, 388) -- code for drawing the bird
love.graphics.setColor(.87, .84, .27) love.graphics.rectangle('fill', 62, 200, 30, 25)
end
If you aren't patient enough to go through the whole steps, you can just paste the following code into your main.lua file. And press CTRL+B.
To revise on use of Sublime:
- Create a folder anywhere in your PC. The folder can have any name.
- Open the folder from Sublime.
- Create a new file named main.lua. (Yes, the name has to be exactly main.lua)
- Copy the following code into main.lua.
- Press CTRL+B.
- VoilĂ !
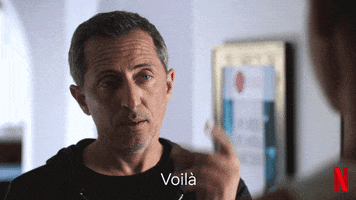
Next: Distribute your game in Windows
Final code
function love.load() playingAreaWidth = 300 playingAreaHeight = 388 birdX = 62 birdWidth = 30 birdHeight = 25 pipeSpaceHeight = 100 pipeWidth = 54 function newPipeSpaceY() local pipeSpaceYMin = 54 local pipeSpaceY = love.math.random( pipeSpaceYMin, playingAreaHeight - pipeSpaceHeight - pipeSpaceYMin ) return pipeSpaceY end function reset() birdY = 200 birdYSpeed = 0 pipe1X = playingAreaWidth pipe1SpaceY = newPipeSpaceY() pipe2X = playingAreaWidth + ((playingAreaWidth + pipeWidth) / 2) pipe2SpaceY = newPipeSpaceY() score = 0 upcomingPipe = 1 end reset() end function love.update(dt) birdYSpeed = birdYSpeed + (516 * dt) birdY = birdY + (birdYSpeed * dt) local function movePipe(pipeX, pipeSpaceY) pipeX = pipeX - (60 * dt) if (pipeX + pipeWidth) < 0 then pipeX = playingAreaWidth pipeSpaceY = newPipeSpaceY() end return pipeX, pipeSpaceY end pipe1X, pipe1SpaceY = movePipe(pipe1X, pipe1SpaceY) pipe2X, pipe2SpaceY = movePipe(pipe2X, pipe2SpaceY) function isBirdCollidingWithPipe(pipeX, pipeSpaceY) return -- Left edge of bird is to the left of the right edge of pipe birdX < (pipeX + pipeWidth) and -- Right edge of bird is to the right of the left edge of pipe (birdX + birdWidth) > pipeX and ( -- Top edge of bird is above the bottom edge of first pipe segment birdY < pipeSpaceY or -- Bottom edge of bird is below the top edge of second pipe segment (birdY + birdHeight) > (pipeSpaceY + pipeSpaceHeight) ) end if isBirdCollidingWithPipe(pipe1X, pipe1SpaceY) or isBirdCollidingWithPipe(pipe2X, pipe2SpaceY) or birdY > playingAreaHeight then reset() end local function updateScoreAndClosestPipe(thisPipe, pipeX, otherPipe) if upcomingPipe == thisPipe and (birdX > (pipeX + pipeWidth)) then score = score + 1 upcomingPipe = otherPipe end end updateScoreAndClosestPipe(1, pipe1X, 2) updateScoreAndClosestPipe(2, pipe2X, 1) end function love.draw() love.graphics.setColor(.14, .36, .46) love.graphics.rectangle('fill', 0, 0, playingAreaWidth, playingAreaHeight) love.graphics.setColor(.87, .84, .27) love.graphics.rectangle('fill', birdX, birdY, birdWidth, birdHeight) local function drawPipe(pipeX, pipeSpaceY) love.graphics.setColor(.37, .82, .28) love.graphics.rectangle( 'fill', pipeX, 0, pipeWidth, pipeSpaceY ) love.graphics.rectangle( 'fill', pipeX, pipeSpaceY + pipeSpaceHeight, pipeWidth, playingAreaHeight - pipeSpaceY - pipeSpaceHeight ) end drawPipe(pipe1X, pipe1SpaceY) drawPipe(pipe2X, pipe2SpaceY) love.graphics.setColor(1, 1, 1) love.graphics.print(score, 15, 15) end function love.keypressed(key) if birdY > 0 then birdYSpeed = -165 end end
Next: Distribute your game in Windows
Comments
Post a Comment
Thanks for the comment! :)